TypeScript
Starting with version 2.2, Kea officially supports TypeScript!
Get type completion when use
ing logic:
... and while writing it:
There's just one gotcha.
TypeScript doesn't support the funky loopy syntax that Kea uses, making impossible to automatically generate types for code like this:
I tried many ways to get this to work, but it was just not possible.
Even if I'd totally change the syntax of Kea itself, several things would
still not be possible be hard to implement or have big limitations
with today's TypeScript. For example selectors that
recursively depend on each other... or plugins.
Check out this blog post for a full overview of all the failed approaches.
Thus a workaround was needed.
kea-typegen
#
Automatic types with kea-typegen
uses the TypeScript Compiler API to
analyse your .ts
files and generate type definitions for your logic.
Run kea-typegen write
or kea-typegen watch
and get a bunch of logicType.ts
files:
The generated types will be automatically added to the kea()
call.
It's a bit of extra work, but works like magic once set up!
#
Step 1. Install packagesFirst install the kea-typegen
and typescript
packages:
#
Step 2. Actually ignore the typesAdd this to your .gitignore
:
Typefiles are generated and used mainly for coding assistance and CI/CD, so it doesn't make sense to pollute commits with them. Plus they will cause merging issues at pull requests. Just ignore them.
If you're transpiling TypeScript via babel
, you won't need to generate any types before a build.
#
Step 3. Run it- While developing, run
kea-typegen watch
, and it'll generate new types every time your logic changes. - Run
kea-typegen write
to generate all the types, for example before a production build. - Finally,
kea-typegen check
can be used to see if any types need to be written
Here's a sample pacakge.json
, that uses concurrently
to run kea-typegen watch
together with webpack while developing and
kea-typegen write
before building the production bundle.
#
Step 4. Specify types for actions and defaultsThe only places you need to specify types are action
parameters and defaults (e.g. for reducers
or loaders
)
Files generated with kea-typegen will automatically import any types they can, and add the rest as type arguments
for kea<logicType<LocalType, LocalUser>>
#
Caveats / Known issues- Using namespaced types like
ExportedApi.RandomThing
is slightly broken. You may sometimes need to create aninterface
thatextends
the original type. Creating atype
alias will not work, as "One difference is that interfaces create a new name that is used everywhere. Type aliases donβt create a new name β for instance, error messages wonβt use the alias name. "
With some tools you might need to "Reload All Files" or explicitly open
logicType.ts
to see the changes.Plugins aren't supported yet. I've hardcoded a few of them (loaders, router, window-values) into the typegen library, yet that's not a long term solution.
logic.extend()
doesn't work yet
Found a bug? Some type wrongly detected? Post an issue here.
#
Different path for types (not recommended)It's possible to write the type files into a different folder as arguments to the kea-typegen
commands
or with a .kearc
file:
However, experience would suggest against doing so. Having the logicType.ts
files in the same folder as the code
helps detect possible coding and typing issues sooner (the files show up red in the sidebar).
#
Alternative: MakeLogicType<V, A, P>At the end of the day, we are forced to make our own logicTypes
and feed them to kea()
calls.
However nothing says these types need to be explicitly made by kea-typegen
.
You could easily make them by hand. Follow the example
and adapt as needed!
To help with the most common cases, Kea 2.2.0 comes with a special type:
Pass it a bunch of interfaces denoting your logic's values
, actions
and props
...
and you'll get a close-enough approximation of the generated logic.
The result is a fully typed experience:
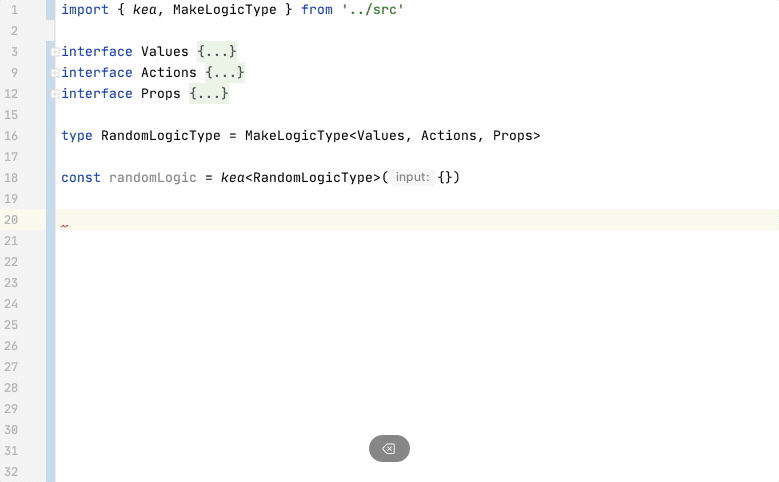
You'll even get completion when coding the logic:
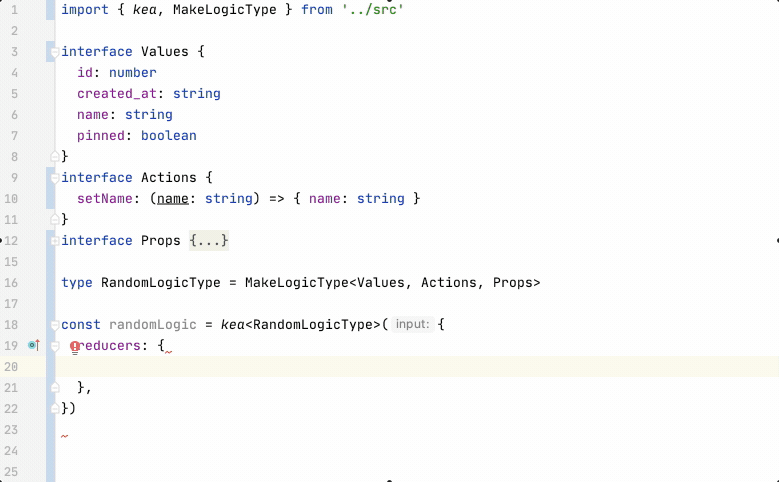
Thank you to the team at Elastic for inspiring this approach!
Next steps
- Read about Debugging to be even more productive when writing Kea code.